OBJECTIVE
The objective of this project is to simulate a rolling die. I will use LEDs arranged in the pattern of a conventional die and give the illusion that it is ‘rolling’ as a number is randomly chosen.
THE CODE
The first thing that I did was to create and initialise an array, with the data type of int. There is more than one way that I could have written this, so I want to show you two different ways of writing it, plus an optimised way (due to how the compiler works and the evolution of C++).
int ledPins[7] = {2, 3, 4, 5, 6, 7, 8};
You could write it this way
int ledPins[] = {2, 3, 4, 5, 6, 7, 8};
You could write it this way. The square brackets can be left empty and the compiler will assume the size based on our 7 values.
int ledPins[] {2, 3, 4, 5, 6, 7, 8, 9};
The evolution of C++ means that you can now remove the equal sign, this is called universal initialisation. This is the line of code that I will use.
TOP TIP
Universal initialisation
Since C++ 11 there has been in existance univeral initilisation.
I then create a dice pattern using a multidimensional array. As with the previous line of code, I do not need an equal sign.
To work out which LEDs need to be ON and when, I created a diagram that designates each LED with a number from 1 – 7. I then constructed a table to plot which LEDs need to be ON for each number. I then took the values of each row and placed them into a multidimensional array.
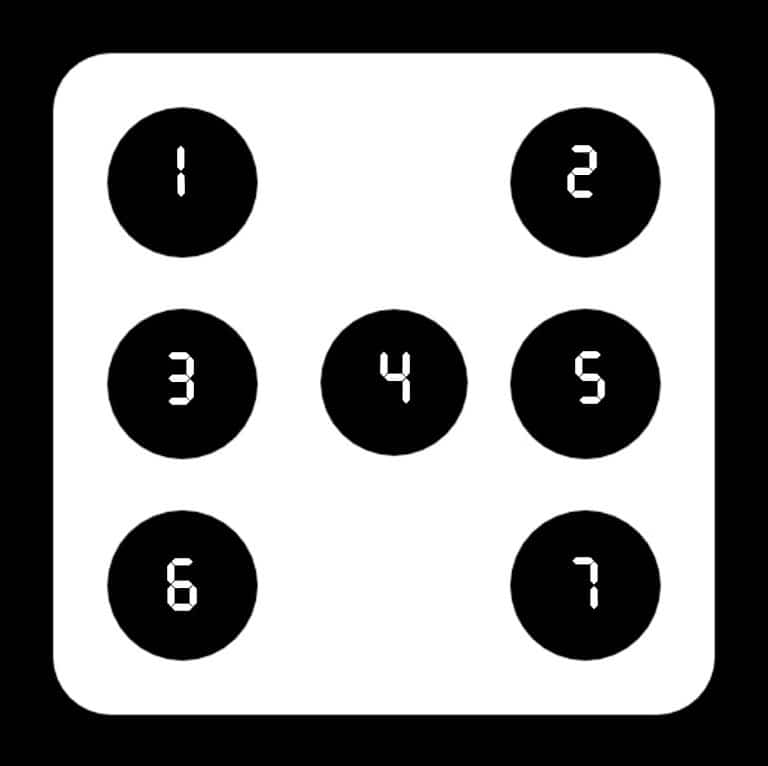
LED 1 | LED 2 | LED 3 | LED 4 | LED 5 | LED 6 | LED 7 | |
POSITION 1 | 0 | 0 | 0 | 1 | 0 | 0 | 0 |
POSITION 2 | 1 | 0 | 0 | 0 | 0 | 0 | 1 |
POSITION 3 | 1 | 0 | 0 | 1 | 0 | 0 | 1 |
POSITION 4 | 1 | 1 | 0 | 0 | 0 | 1 | 1 |
POSITION 5 | 1 | 1 | 0 | 1 | 0 | 1 | 1 |
POSITION 6 | 1 | 1 | 1 | 0 | 1 | 1 | 1 |
int dicePatterns[7][7] = {
{0, 0, 0, 0, 0, 0, 1}, // POSITION 1
{0, 0, 1, 1, 0, 0, 0}, // POSITION 2
{0, 0, 1, 1, 0, 0, 1}, // POSITION 3
{1, 0, 1, 1, 0, 1, 0}, // POSITION 4
{1, 0, 1, 1, 0, 1, 1}, // POSITION 5
{1, 1, 1, 1, 1, 1, 0}, // POSITION 6
{0, 0, 0, 0, 0, 0, 0} // NO NUMBER
};
Next I define 2 variables, with the data type of int. I set the switch to use pin 9 on the Arduino.
int switchPin = 9; //Define button pin
int blank = 6;
In the setup() I created a for loop
In the loop() I created an if statement. I then added a 100ms delay.
I then created a function called rollTheDice(), which contains two for loops. I then created a function called show.